Innovate
Your Software
Faster without Risk
Innovate Your Software Faster without Risk
How to Implement Feature Flags in Python
Feature flags, also known as feature toggles, are a powerful tool in a developer's arsenal. They allow you to enable or disable functionalities within your application without deploying new code.
Here's a breakdown of the key benefits of using feature flags:
- Safe Deployments: Release new features gradually to a specific user group or percentage of users before a full rollout.
- A/B Testing: Easily compare different versions of a feature by directing users to variations controlled by feature flags.
- Feature Rollbacks: Quickly disable buggy or unintended features without a new deployment.
- Configuration Management: Manage application behavior through flags instead of hardcoded values.
In this article, we'll explore how to implement feature flags in Python using FeatBit, a popular open-source solution. We'll walk through defining our feature flags, creating a simple Flask application, and integrating the FeatBit client.
Create a Flask App
To showcase feature flags, we use Flask to create a simple web app. To start, we create a folder for our app named demo, create a file named demo.py in that folder and install Flask in using pip install Flask
.
In demo.py, create a home route that returns a basic "Hello, World!" and a /users/<user>
route that returns the user string:
from flask import Flask
app = Flask(__name__)
@app.route("/")
def hello_world():
return "<p>Hello, World!</p>"
@app.route("/users/<user>", methods=['GET'])
def show_user(user: str):
return f"<p>Hello, {user}!</p>"
Finally run flask --app demo run and go to http://127.0.0.1:5000 to see your basic app running.
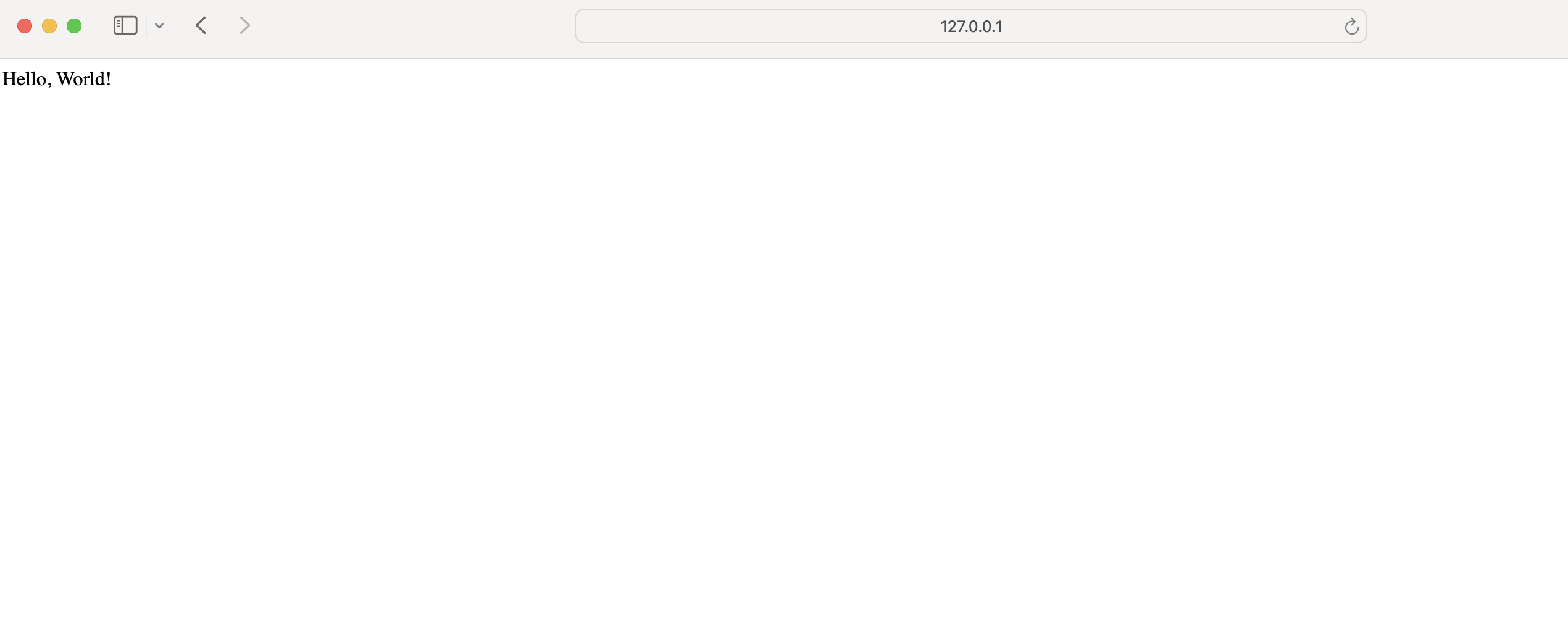
Create our feature flags
FeatBit (as an open source feature flags management tool) offers a lightweight and easy-to-use library for managing feature flags. It provides a client-side SDK that interacts with a FeatBit server, allowing you to control feature availability remotely.
Run FeatBit Server
To self-host FeatBit with Docker, Run this script:
git clone https://github.com/featbit/featbit
cd featbit
docker compose up -d
Once all containers have started, you can access FeatBit's portal at http://localhost:8081 and log in with the default credentials:
- Username: test@featbit.com
- Password: 123456
Add a feature flag
We are now ready to create and set up our flag. To do this, go to the "Feature Flags" tab in FeatBit's portal and click "Add" Add a boolean type feature flag (we use new-cool-feature
as key), set a target rule where keyId
starting with user-test
returns true
, add a default rule 50% of users returns true
, add any other details and press "Save."
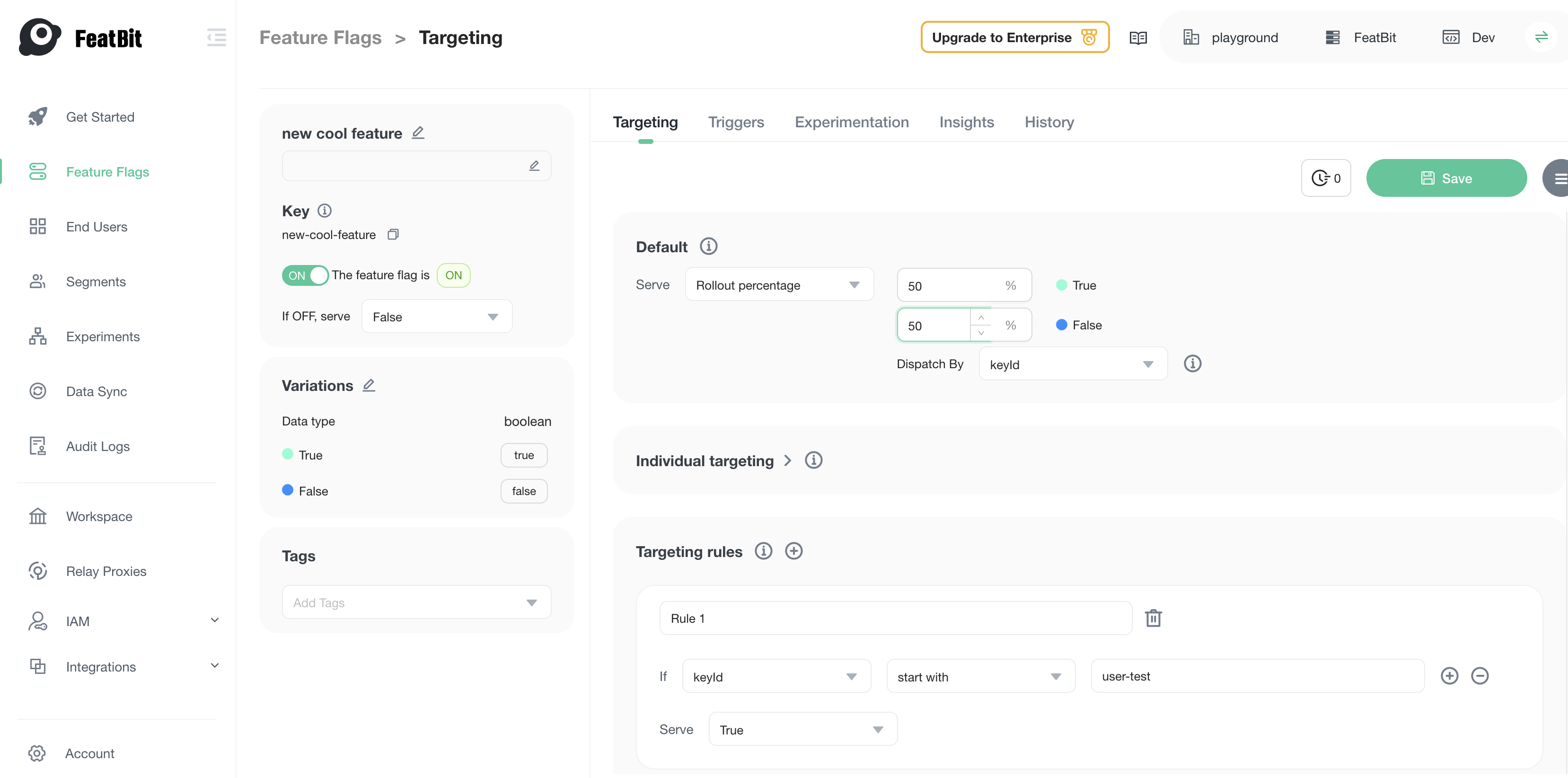
The target rule enables us to test the active feature flag by going to /user/{user_id} route first but is optional. The defaut rule enables us to serve a flag value if no other rule matches(we use rollout percentage in our feature flag). If the flag is offline, a 'False' flag value is predefined.
Integrate FeatBit
To add Featbit in our app, we install python SDK using pip install fb-python-sdk
.
We initialize FeatBit to the demo.py using our environment Secret and local address from project settings. In our user route, we add a check with FeatBit of our new-cool-feature flag. If it is true, we return a different value. If it isn’t, nothing change in the returning value.
from fbclient import get, set_config
from fbclient.config import Config
from flask import Flask
app = Flask(__name__)
env_secret = "your-env-secret"
event_url = "http://localhost:5100"
streaming_url = "ws://localhost:5100"
set_config(Config(env_secret, event_url, streaming_url))
fb_client = get()
@app.route("/")
def hello_world():
return "<p>Hello, World!</p>"
@app.route("/users/<user>", methods=['GET'])
def show_user(user: str):
fb_user = {"key": user, "name": user}
if fb_client.variation("new-cool-feature", fb_user, False):
return f"<p>Welcome, {user}! You have a new cool feature!!!</p>"
else:
return f"<p>Hello, {user}!</p>"
Then we visited /users/user
start with user-test
we get the new content on the page; if user
doesn't start with user-test
, we have a 50% chance to get the new content on the page.
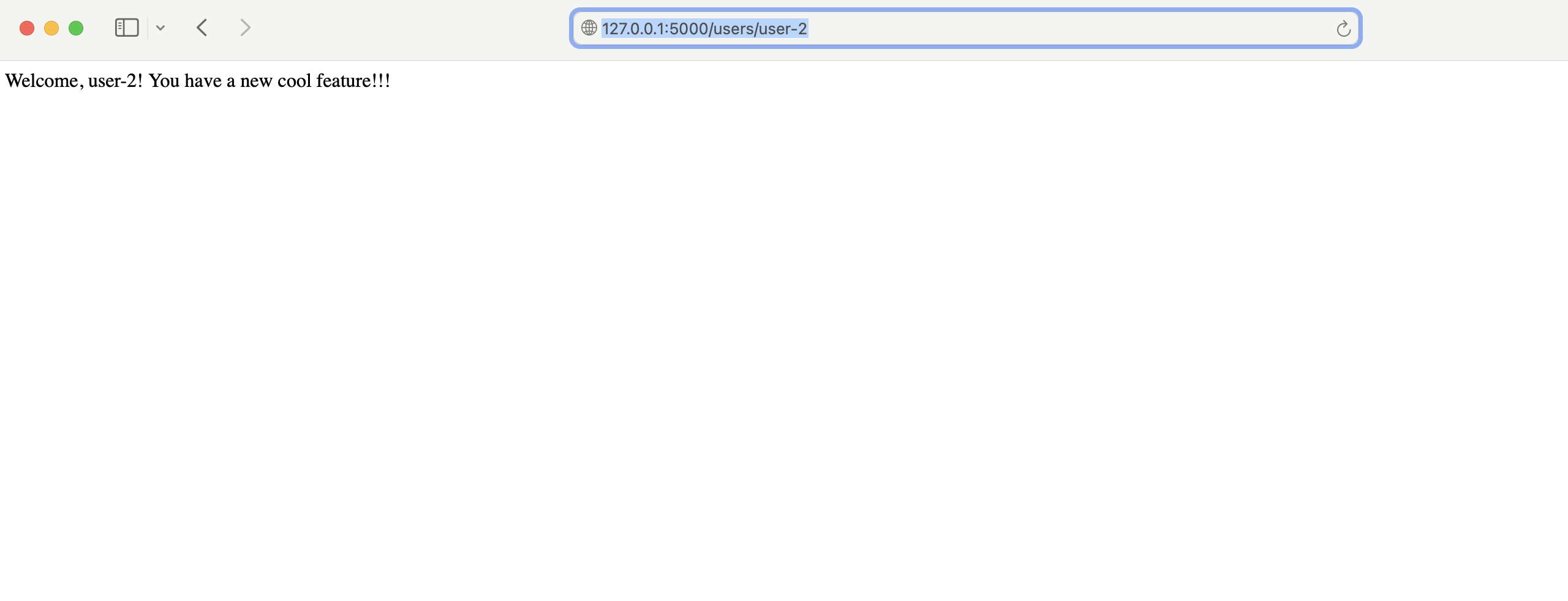
Conclusion
In conclusion, integrating feature flags into Python applications with FeatBit offers developers a seamless way to manage feature releases effectively. By leveraging FeatBit's capabilities, developers can streamline the development process, iterate rapidly, and deliver superior user experiences with confidence. Feature flags, coupled with FeatBit's intuitive interface, empower developers to navigate the complexities of software development with agility and precision.
For further reading, you can get more details in FeatBit docs